ํฐ์คํ ๋ฆฌ ๋ทฐ
[Udemy] JavaScript: The Advanced Concepts - ์น์ 15:Appendix II: Intermediate Javascript
Carrot๐ฅ 2020. 8. 13. 04:30** โ priv | ๊ฐ์๋
ธํธ
์นดํ
๊ณ ๋ฆฌ์ ์๋ ๋ด์ฉ์ ๋ฐํ์ด ๋ชฉ์ ์ด ์๋๊ธฐ ๋๋ฌธ์ ์ ํ ์ ์ ๋์ด์์ง ์์ต๋๋น.**
์น์ 15:Appendix II: Intermediate Javascript
207. Advanced Functions
Closures
a function ran. the function executed. Itโs never going to execute again.
BUT itโs going remember that there reference to those variables.
so the child scope always access to the parent scope.
Currying
const multiply = (a,b) => a*b;
const curriedMultiply = (a) => (b) => a*b;
curriedMultiply(3); // this part returns a function "(b) => 3*b"
Compose
const compose = (f,g) => (a) => f(g(a));
const sum = (num) => num + 1;
compose(sum, sum)(5); // result : 7
+
Avoiding Side Effects, functional purity!
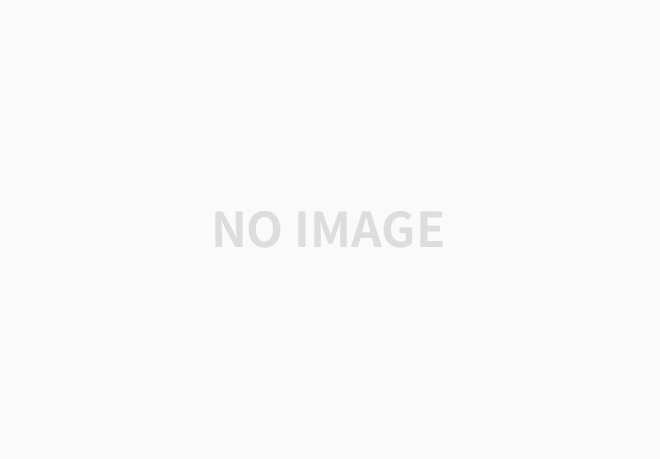
avoiding side effects and always returning we create something that we call deterministic .
deterministic - ๊ฒฐ์ ๋ก ์
๊ฐ์ input์ด๋ฉด ํญ์ same value ๋ฅผ ๋ฆฌํดํ๋ค.
It's a very important concept and that's a key principle in avoiding bugs.
208. Advanced Arrays
square brackets []
map
, filter
, reduce
pure simple functions
It always returns of value.
And there are no side effects.
weโre not changing the array.
just making a new copy of the array.
never mutating the data
209. Advanced Objects
-
reference type
-
context
vsscope
-
instantiation
class Player {
constructor(name, type) {
console.log("Player ", this);
this.name = name;
this.type = type;
}
introduce() {
console.log(`Hi I am ${this.name}, I am a ${this.type}`);
}
}
class Wizard extends Player {
constructor(name, type) {
//console.log("Wizard ", this); // ์ด๋ ๊ฒ์ฐ๋ฉด ์๋ฌ๋จ
// Uncaught ReferenceError: Must call super constructor in derived class before accessing 'this' or returning from derived constructor
super(name, type);
console.log("Wizard ", this);
}
play() {
console.log(`WEEEEEE I'm a ${this.type}`);
}
}
const wizard1 = new Wizard("Judy", "Dark Wizard");
const wizard2 = new Wizard("Nick", "Healer");
wizard1.play(); // WEEEEEE I'm a Dark Wizard
wizard2.introduce(); // Hi I am Nick, I am a Healer
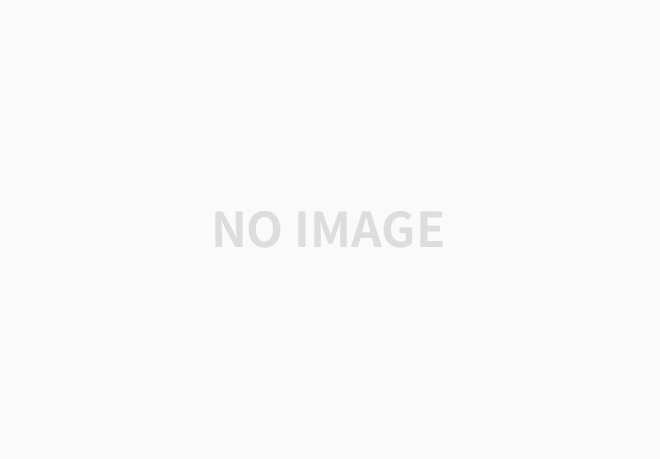
Uncaught ReferenceError: Must call super constructor in derived class before accessing 'this' or returning from derived constructor
ํ์๋ ํด๋์ค์์ this ์ ์ ๊ทผํ๋ ค๋ฉด
super ์์ฑ์๋ฅผ ๋จผ์ ํธ์ถํด์ผ์ง๋ง ๊ฐ๋ฅํ๋ค.
// classical inheritance
var Player = function(name, type) {
this.name = name;
this.type = type;
}
Player.prototype.introduce = function {
console.log(`Hi I am ${this.name}, I am a ${this.type}`);
}
var wizard1 = new Player("Judy", "Dark Wizard");
var wizard2 = new Player("Nick", "Healer");
wizard1.play = function(){
console.log(`WEEEEEE I'm a ${this.type}`);
};
wizard2.play = function(){
console.log(`WEEEEEE I'm a ${this.type}`);
};
210. ES7
ES7์ ๋ณ๊ฑฐ ์์
ES6๋ ๋ญ๊ฐ ๋ง์ด ๋ฐ๋์์๋๋ฐ ES7์ ๋๊ฐ ์ถ๊ฐ๋
-
.includes()
method
added on string and array
const pets = ["cat", "dog", "bat"];
pets.includes("dog"); // true
pets.includes("bird"); // false
"Hello".includes("o"); // true
"Hello".includes("a"); // false
-
exponential operator
const square = (x) => x**2;
square(2); // 4
square(5); // 25
const cube = (y) => y**3;
cube(3); // 27
cube(4); // 64
console.log(10 ** -2); // 0.01
The exponentiation operator (**
) returns the result of raising the first operand to the power of the second operand.
211. ES8
ES7๋ณด๋ค๋ ์ข๋๋ฐ๋๊ธดํจ
์ค์ํ ๋ช๊ฐ๋ง ์ดํด๋ด
-
padStart
str.padStart(targetLength [, padString])
.padEnd()
'abc'.padStart(10); // " abc"
'abc'.padStart(10, "foo"); // "foofoofabc"
'abc'.padStart(6,"123465"); // "123abc"
'abc'.padStart(8, "0"); // "00000abc"
'abc'.padStart(1); // "abc"
-
Trailing commas in functions
ECMAScript 2017 allows trailing commas in function parameter lists.
the ending comma is now valid
function f(p) {}
function f(p,) {}
(p) => {};
(p,) => {};
์๋๋ ๋ฐฐ์ด๋ฆฌํฐ๋ด์์๋ง ํ์ฉ๋์๊ณ ,
ES5์์ ๊ฐ์ฒด๋ฆฌํฐ๋ด์์๋ ํ์ฉ๋จ,
ES7๋ถํฐ ํจ์์ ํ๋ผ๋ฏธํฐ์๋ ํ์ฉ๋จ
https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Trailing_commas
-> ๋ฒ์ ๊ด๋ฆฌ ์ด๋ ฅ์ด ๊ฐ๋จํด์ง๊ณ ์ฝ๋ ํธ์ง์ด ๋ ํธํด์ง๋ค๋ ์ฅ์ ์ด ์์ต๋๋ค.
-
Object.values
,Object.entries
Object.values : ๊ฐ์ฒด๊ฐ ๊ฐ์ง๋ (์ด๊ฑฐ ๊ฐ๋ฅํ) ์์ฑ์ ๊ฐ๋ค๋ก ์ด๋ฃจ์ด์ง ๋ฐฐ์ด์ ๋ฆฌํดํฉ๋๋ค.
Object.entries : ๊ฐ์ฒด๊ฐ ๊ฐ์ง๋ (์ด๊ฑฐ ๊ฐ๋ฅํ) ์์ฑ์ [key, value]
์์ ๋ฐฐ์ด์ ๋ฐํํฉ๋๋ค.
Obj ๋ฅผ ๋ฐฐ์ด๋ก ๋ณํํด์ ์ฌ์ฉ
let obj = {
username0 : "Santa",
username1 : "Rudolf",
username2 : "Mr.Grinch",
}
Object.keys(obj).forEach((key, index) => {
console.log(key, obj[key], index);
});
// username0 Santa 0
// username1 Rudolf 1
// username2 Mr.Grinch 2
Object.values(obj).forEach((value) => {
console.log(value);
});
// Santa
// Rudolf
// Mr.Grinch
Object.entries(obj);
// [
// ["username0", "Santa"],
// ["username1", "Rudolf"],
// ["username2", "Mr.Grinch"]
// ]
Object.entries(obj).map(val => val[1]+val[0].replace("username", ""));
//["Santa0", "Rudolf1", "Mr.Grinch2"]
213. ES10 (ES2019)
arr.flat()
nested Array -> flat Array (์ค์ฒฉ๋ฐฐ์ด ํํํ)
[1,[2,3],[4,5]].flat()
// [ 1,2,3,4,5 ]
[1, 2,[3,4,[5]]].flat()
// [ 1, 2, 3, 4, [5] ]
[1, 2,[3,4,[5]]].flat(2)
// [1, 2, 3, 4, 5]
arr.flat(depth)
depth๊ธฐ๋ณธ๊ฐ์ 1
https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/flat
const arr5 = [1, 2, , , 5, 6];
arr5.flat();
// [1, 2, 5, 6] ๋ฐฐ์ด์ ๊ตฌ๋ฉ๋ ์ ๊ฑฐ
// Turn the trapped 3 number into: [3]
const trapped = [[[[[[[[[[[[[[[[[[[[[[[[[[3]]]]]]]]]]]]]]]]]]]]]]]]]];
//Solution
console.log(trapped.flat(Infinity))
// Infintiy is actually a LARGE number in JavaScipt.
// It represents the maximum amount of memory that we can hold for a number!
// Learn more here:
// https://riptutorial.com/javascript/example/2337/infinity-and--infinity
const jurassicPark = [
["๐ฆ", "๐ฆ"],
"๐",
"๐ข",
[["๐", "๐ฆ"]],
[[[["๐ฆ"]]]],
["๐", "๐ฆ"]
];
jurassicPark.flat(50);
// (9) ["๐ฆ", "๐ฆ", "๐", "๐ข", "๐", "๐ฆ", "๐ฆ", "๐", "๐ฆ"]
arr.flatMap()
์ด๋ ๊น์ด 1์ flat ์ด ๋ค๋ฐ๋ฅด๋ map ๊ณผ ๋์ผํ์ง๋ง, flatMap ์ ์์ฃผ ์ ์ฉํ๋ฉฐ ๋์ ํ๋์ ๋ฉ์๋๋ก ๋ณํฉํ ๋ ์กฐ๊ธ ๋ ํจ์จ์ ์ ๋๋ค.
const jurassicParkChaos = jurassicPark.flatMap(
creature => creature + "๐ฆ"
);
// jurassicParkChaos
//(6) ["๐ฆ,๐ฆ๐ฆ", "๐๐ฆ", "๐ข๐ฆ", "๐,๐ฆ๐ฆ", "๐ฆ๐ฆ", "๐,๐ฆ๐ฆ"]
str.trimStart()
const userEmail1 = " judy@email.com";
const userEmail2 = "nick@email.com ";
userEmail1.trimStart();
// "judy@email.com"
userEmail2.trimEnd();
// "nick@email.com"
Object.fromEntries
const inventory = [
["potato", 23],
["apple", 40],
["broccoli", 15]
];
const obj = Object.fromEntries(inventory);
// {potato: 23, apple: 40, broccoli: 15}
Object.entries(obj);
// ์ด๋ ๊ฒ ํ๋ฉด inventory๋ ๊ฐ์ ๋ชจ์์ผ๋ก ๋ค์ ๋ฐ๋
// ๊ธ๊ป fromEntries <-> entries ์ด๋ฐ ๋ฌ๋
try
& catch
catch(error) ์์ error ํ๋ผ๋ฏธํฐ ์๋ต๊ฐ๋ฅ
214. Advanced Loops
for of
iterating - array, strings
we are able to iterate over individual items
for (item of obj){ console.log(item);}
// Uncaught TypeError: obj is not iterable
for in
- properties
enumerating - object
enumerable
( enumerate : ์ด๊ฑฐํ๋ค)
it allows us to see the property
216. Modules
code reusablility
global namespace
dependency resolution
Inline Script -> Script Tags -> IIFE ->
Common JS + browserify
// js1
module.exports = function add (a,b){
return a + b;
};
// js2
var add = require("./add");
module bundler
ES6 + Webpack2
// js1
export const add = (a,b) => A + b;
// or
export default function add() {
return a + b;
}
// js2
import { add } from "./add";
// or
import add from "./add";
์ถ์ฒ : Udemy - JavaScript: The Advanced Concept
https://www.udemy.com/course/advanced-javascript-concepts
'๊ณต๋ถ > priv | ๊ฐ์๋ ธํธ' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
- ๋ฐ๋๋ผ์ฝ๋ฉ ํ๊ธฐ
- DOM
- HTML
- ์ฝ๋ฉ๋ถํธ์บ ํ
- js
- css
- ์์ฑ์ํจ์
- string
- KEYBOARD
- Stash
- ๋ถํธ์บ ํ
- review
- ๋ฐ๋๋ผ์ฝ๋ฉ
- eslint
- array
- eventlistener
- book
- VSC
- stackoverflow
- GIT
- Total
- Today
- Yesterday